Special elements
21/09/2024 by zavos.dev
Hello there, fellow Svelte enthusiasts!
Svelte introduces unique elements that enhance component-based development.
These
special elements provide powerful mechanisms for manipulating component context and
dynamically rendering other components, offering greater control and flexibility in your
Svelte applications.
<svelte:self>
- README.md
<svelte:component>
A component can change its type altogether with
<svelte:component>
Red thing
+page.svelte
<select bind:value={selected}> {#each options as option} <option value={option}>{option.color}</option> {/each} </select> <svelte:component this={selected.component} />
<svelte:element>
As with the previous exercise, we can replace a long sequence of if blocks with a single dynamic
element
You have successfully selected h1.
+page.svelte
<svelte:element this={selected2}> <p class="text-2xl font-semibold">You have successfully selected {selected2}. </p> </svelte:element>
<svelte:window>
Just as you can add event listeners to any DOM element, you can add event listeners to the window
object with <svelte:window>.
Focus this window and press any key
+page.svelte
<svelte:window on:keydown={handleKeyDown} />
<svelte:window> bindings
svelte:window bindings provide a convenient way to react to events that occur on the browser window. This includes events like resizing, scrolling, or changes in focus.
You have scrolled undefined pixels
+page.svelte
<svelte:window bind:scrollY={y} on:keydown={handleKeyDown} />
svelte:body
Similar to svelte:window, the svelte:body element allows you to listen for events
that fire on document.body. This is useful with the mouseenter and mouseleave events,
which don't fire on window.
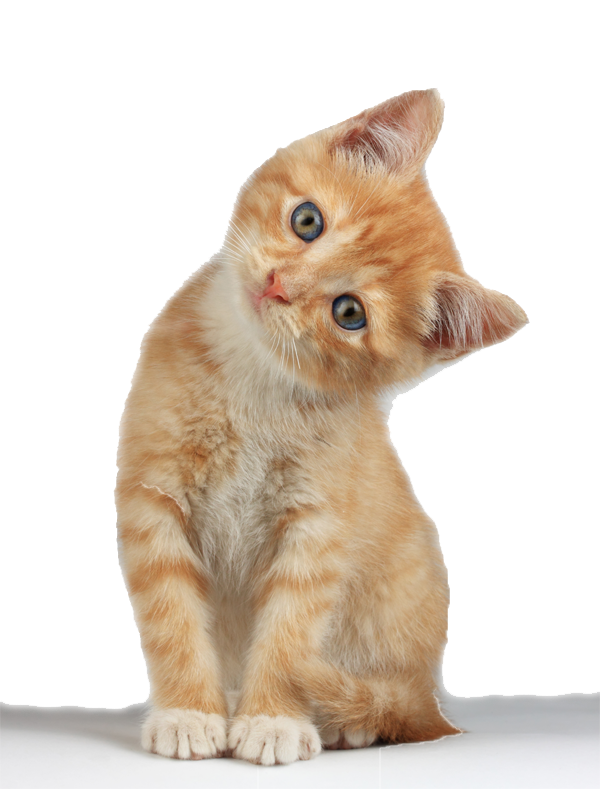
Move your cursor away from the site
+page.svelte
<svelte:body on:mouseenter={() => (hereKitty = true)} on:mouseleave={() => (hereKitty = false)} />
svelte:document
The svelte:document element allows you to listen for events that fire on document. This
is useful with events like selectionchange, which doesn't fire on window.
Highlight this text in order to select it below
Selection:
+page.svelte
<svelte:document on:selectionchange={handleSelectionChange} />
svelte:head
The svelte:head element allows you to insert elements inside the head of your
document. This is useful for things like title and meta tags, which are critical for
good SEO.
+page.svelte
<div class="flex gap-2"> <input class="input bg-slate-900" type="text" bind:value={inputText} placeholder="Type a new title here" /> <button class="btn" on:click={updateTitle(inputText)}>Update title</button> </div> <svelte:head> <title>{title}</title> </svelte:head>
svelte:options
The svelte:options element allows you to specify compiler options such as
immutable and accessors.
todos
svelte:fragment
svelte:fragment is a powerful tool in Svelte for grouping elements together without introducing
an additional element in the DOM. This can improve performance and readability, especially when
dealing with complex components or conditional rendering.